Laravel Observer: Automatically Triggered Actions When Data Changes
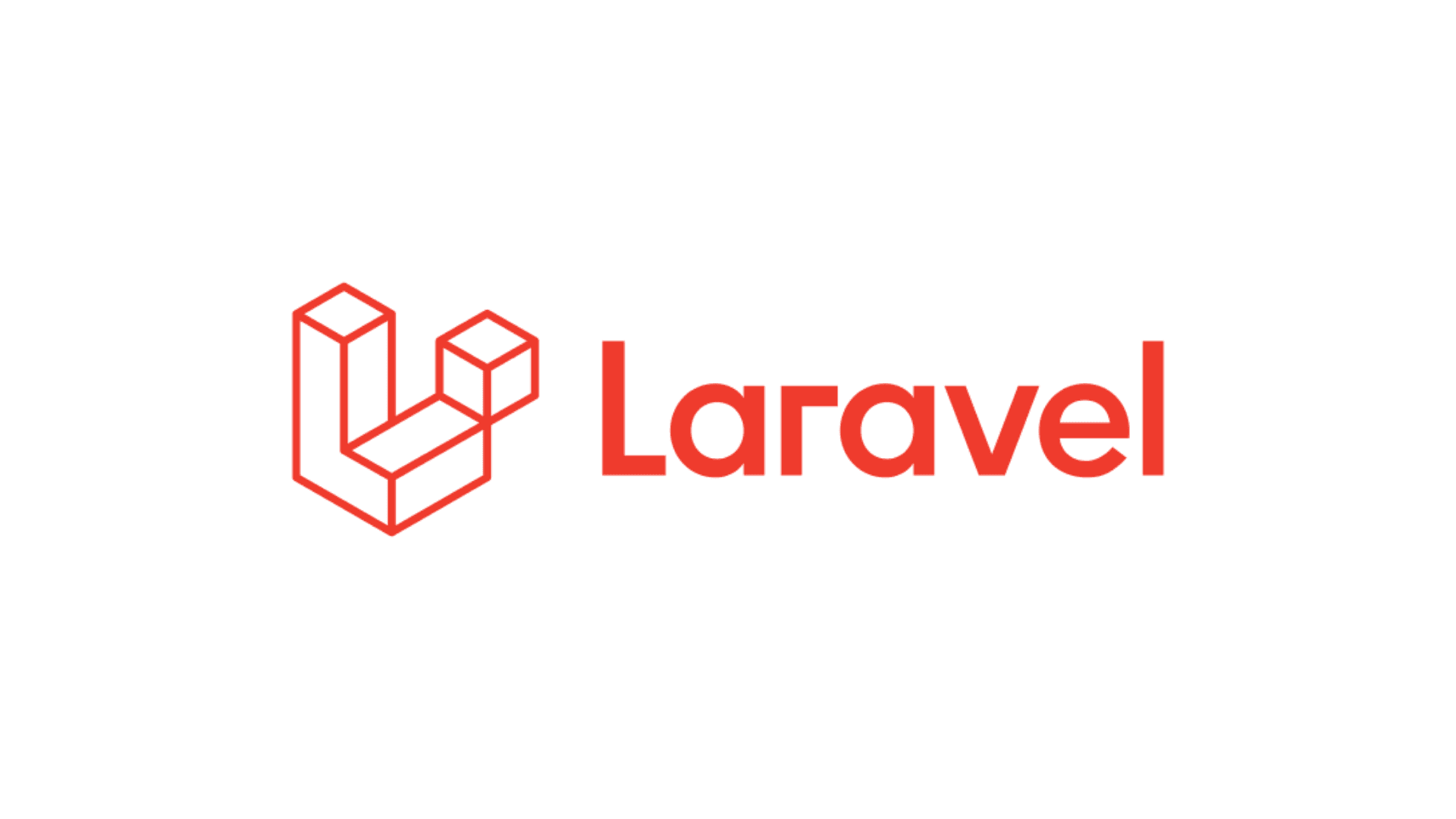
Use Case: Automatically Generating Slugs for Posts
In web application development, there are many cases where we want to automatically trigger actions when data is created, updated, or deleted. One of the cleanest and most elegant ways to do this in Laravel is by using an Observer.
In this article, we'll discuss how to automatically generate a slug every time a Post is created or updated using a Laravel Observer.
What is a Laravel Observer?
A Laravel Observer is a class that allows you to "observe" model events and run specific logic when events such as creating
, updating
, deleting
, etc. occur.
This is particularly useful for separating logic from your models or controllers, keeping your code cleaner and more organized.
Use Case: Auto-Generating Slugs
Let's say we have a posts
table with the following columns:
title
content
slug
We want the slug
to be automatically generated from the title
whenever a post is saved to the database.
Example:
Title: Learning Laravel Observer
Slug: learning-laravel-observer
1. Creating the Model and Migration
Generate the Post
model and its migration:
php artisan make:model Post -m
Edit the migration file:
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('content');
$table->string('slug')->unique();
$table->timestamps();
});
Then run the migration:
php artisan migrate
2. Creating the Observer
Generate the observer with the following command:
php artisan make:observer PostObserver --model=Post
Add the slug generation logic inside the observer:
namespace App\Observers;
use App\Models\Post;
use Illuminate\Support\Str;
class PostObserver
{
public function creating(Post $post)
{
$post->slug = Str::slug($post->title);
}
public function updating(Post $post)
{
$post->slug = Str::slug($post->title);
}
}
The
Str::slug()
helper from Laravel converts a string into a URL-friendly slug.
3. Registering the Observer
Open AppServiceProvider.php
and register the observer inside the boot()
method:
use App\Models\Post;
use App\Observers\PostObserver;
public function boot(): void
{
Post::observe(PostObserver::class);
}
4. Testing It Out
Try creating a post from a controller, Tinker, or a seeder:
Post::create([
'title' => 'Laravel Observer Is Very Helpful',
'content' => 'This is the body content...'
]);
The slug will automatically be generated:
laravel-observer-is-very-helpful
Visual Workflow
Here is an illustration of how the observer works in Laravel:
Note: This image is illustrative. You can replace it with a custom diagram to show the event flow.
Benefits of Using Observers
- Keeps business logic out of the controller or model
- More modular and maintainable code
- Easy to test and reuse
- Ideal for use cases like audit trails, change logs, and automated processing
Conclusion
Laravel Observers provide a clean and efficient way to automatically trigger actions on model events. In this example, we implemented automatic slug generation when a post is created or updated.
You can take it a step further by validating slug uniqueness or adding suffixes if a duplicate is found.