Getting to Know Laravel Eloquent: An Easy Way to Work with Databases
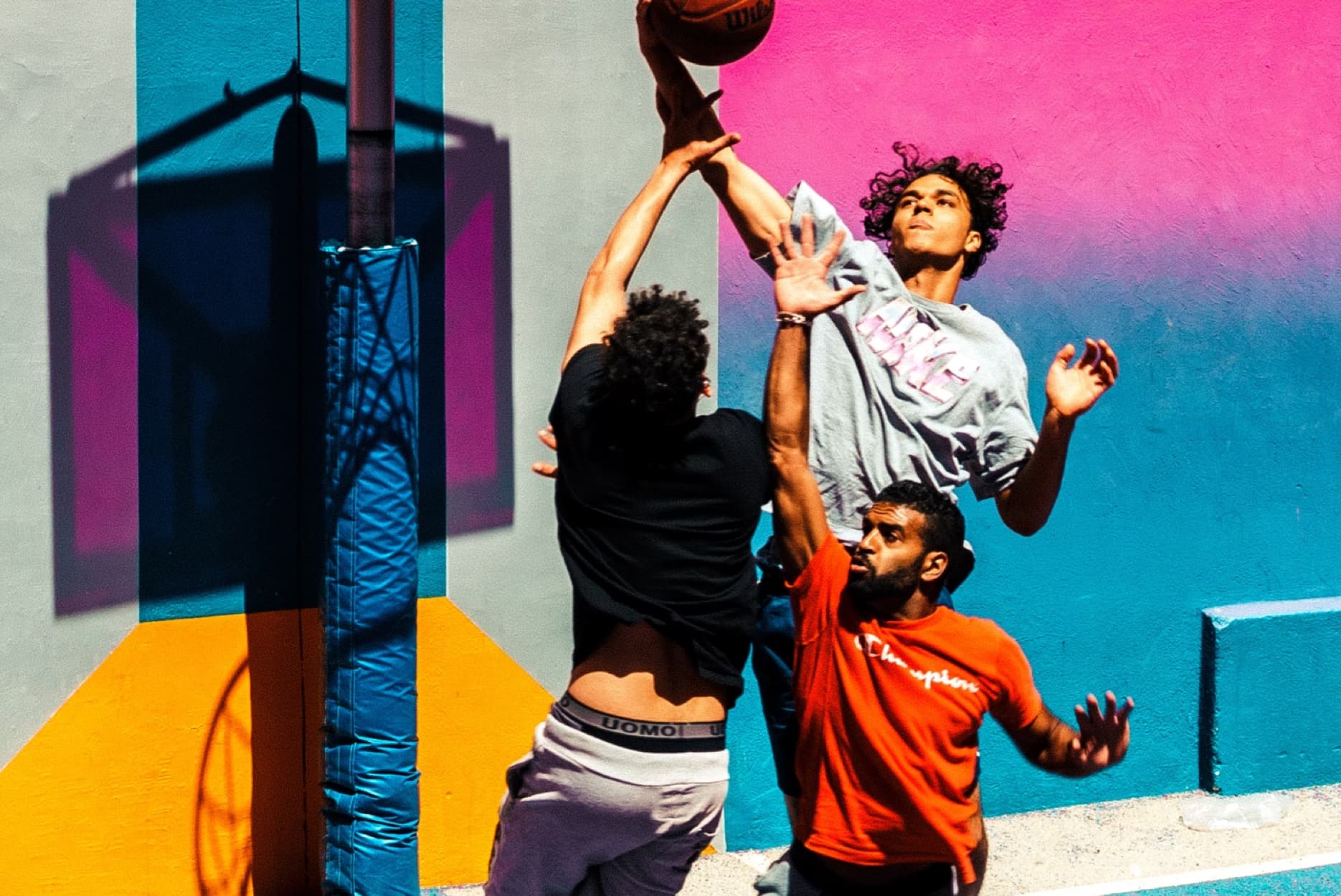
When building web applications, one of the most essential components is how you interact with the database. Laravel, one of the most popular PHP frameworks today, offers Eloquent ORM—a powerful and elegant way to work with databases using object-oriented syntax.
In this post, we'll introduce you to the basics of Laravel Eloquent, how it works, and why it's so developer-friendly.
What is Eloquent?
Eloquent is Laravel’s built-in Object-Relational Mapper (ORM). It allows you to interact with your database tables as if they were PHP classes.
Each table in your database has a corresponding "Model" that allows you to query for data, insert new records, update existing ones, and even define relationships between tables—all using a clean and fluent API.
Setting Up a Model
To create a model in Laravel, you can use the Artisan command:
php artisan make:model Post
This will create a Post
model in the app/Models
directory.
By default, Eloquent assumes the table name is the plural form of the model (posts
for the Post
model), but you can customize it by setting the $table
property.
class Post extends Model
{
protected $table = 'blog_posts';
}
Basic CRUD with Eloquent
Create
$post = new Post;
$post->title = 'Hello World';
$post->content = 'This is my first blog post.';
$post->save();
Or use mass assignment:
Post::create([
'title' => 'Hello World',
'content' => 'This is my first blog post.',
]);
Make sure to add
protected $fillable
in your model for mass assignment.
protected $fillable = ['title', 'content'];
Read
$posts = Post::all(); // Get all posts
$post = Post::find(1); // Find post with ID 1
Update
$post = Post::find(1);
$post->title = 'Updated Title';
$post->save();
Delete
$post = Post::find(1);
$post->delete();
Using Query Scopes
Eloquent lets you define reusable query logic using local scopes:
public function scopePublished($query)
{
return $query->where('is_published', true);
}
Then call it like this:
$posts = Post::published()->get();
Eloquent Relationships
Eloquent makes it super easy to define relationships:
One to Many
// In User model
public function posts()
{
return $this->hasMany(Post::class);
}
Belongs To
// In Post model
public function user()
{
return $this->belongsTo(User::class);
}
You can now do things like:
$user = User::find(1);
$posts = $user->posts;
$post = Post::find(1);
$author = $post->user;
Final Thoughts
Eloquent is one of the reasons Laravel is so enjoyable to work with. It abstracts the complexity of raw SQL and gives you a clean, expressive syntax to define models, query data, and manage relationships.
Whether you're building a small blog or a full-scale web application, mastering Eloquent will significantly boost your productivity and code quality.
Happy coding! 🚀
Got questions about Eloquent or Laravel in general? Drop them in the comments or reach out to me on LinkedIn or GitHub.